Superelevation creator
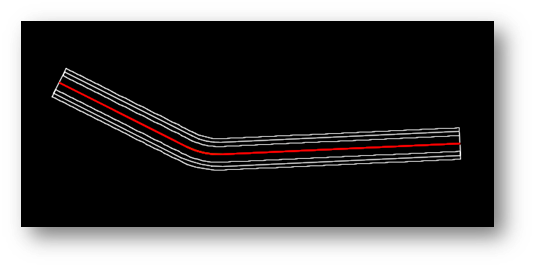
Description
- This is a custom interactive tool for creating superelevation sections and lanes over an alignment.
- The user selects a horizontal alignment.
- The SuperElevationCreator class which extends DgnElementSetTool which handles different events to interact with UI, the SuperElevationCreator class overrides the events here in this tool.
Remarks
- This sample code is a part of ManagedSDKExample which you get with SDK installation under "examples" section in SDK installation directory.
- If you encounter any error while using DgnElementSetTool class, make sure to add a reference to Bentley.DgnDisplayNet.dll by selecting Project > Add Reference or change the projects .csproj file to add reference to this dll.
- The default dll location will be "C:\Program Files\Bentley\OpenRoads Designer CE 10.11\OpenRoadsDesigner\Bentley.DgnDisplayNet.dll".
Source Code
//Required References
using System.Collections.Generic;
using System;
using Bentley.DgnPlatformNET;
using Bentley.DgnPlatformNET.Elements;
using Bentley.CifNET.SDK.Edit;
using Bentley.CifNET.GeometryModel.SDK;
using Bentley.CifNET.GeometryModel.SDK.Edit;
namespace ManagedSDKExample
{
class SuperElevationCreator : DgnElementSetTool
{
/*----------------------------------------------------------------------------------------------**/
/* Write Function | The user is prompted to select a horizontal alignment.
/* This tool creates superelevation section and superlevation lanes left and right.
/*--------------+---------------+---------------+---------------+---------------+----------------*/
protected override void OnPostInstall()
{
base.BeginPickElements();
AccuSnap.LocateEnabled = true;
AccuSnap.SnapEnabled = false;
base.OnPostInstall();
NotificationManager.OutputPrompt("Select a horizontal alignment.");
BeginDynamics();
}
protected override bool OnPostLocate(HitPath path, out string cantAcceptReason)
{
//checks that hitpath is not null
if (path == null)
{
cantAcceptReason = "HitPath is null.";
return false;
}
//checks that the cursor element is not null
Element el = path.GetCursorElement();
if (el == null)
{
cantAcceptReason = "There is no element at cursor.";
return false;
}
//checks that the cursor element is a civil element
if (el.ElementType != MSElementType.Line && el.ElementType != MSElementType.LineString && el.ElementType != MSElementType.ComplexString)
{
cantAcceptReason = "This is not a civil element.";
return false;
}
Bentley.CifNET.SDK.ConsensusConnection con = ConsensusConnectionEdit.GetActive();
if (con == null)
{
cantAcceptReason = "There was an error connecting to the Civil SDK model.";
return false;
}
Alignment al = (el.ParentElement == null) ? Alignment.CreateFromElement(con, el) : Alignment.CreateFromElement(con, el.ParentElement);
if (al == null)
{
cantAcceptReason = "This is not a civil element.";
return false;
}
cantAcceptReason = String.Empty;
return true;
}
public static void InstallNewInstance()
{
SuperElevationCreator cmd = new SuperElevationCreator();
cmd.InstallTool();
}
protected override bool OnDataButton(Bentley.DgnPlatformNET.DgnButtonEvent ev)
{
HitPath hitPath = DoLocate(ev, true, 1);
if (hitPath == null)
return false;
Element element = hitPath.GetHeadElement();
if (element == null)
return false;
FeatureDefinitionManager fdm = FeatureDefinitionManager.Instance;
ConsensusConnectionEdit con = ConsensusConnectionEdit.GetActive();
IEnumerable<string> featureNames = fdm.GetFeatureDefinitions(con, "SuperElevation");
Bentley.CifNET.GeometryModel.SDK.Alignment alignment = Bentley.CifNET.GeometryModel.SDK.Alignment.CreateFromElement(con, element);
if (alignment == null)
{
NotificationManager.OutputPrompt("This is not a horizontal alignment.");
return false;
}
double alignmentLength = alignment.LinearGeometry.Length;
CreateSuperElevations(alignment);
EndDynamics();
NotificationManager.OutputPrompt("Command complete. Select a new horizontal alignment or pick element selection tool to exit command.");
return true;
}
protected override bool OnResetButton(DgnButtonEvent ev)
{
InstallNewInstance();
return true;
}
protected override void OnRestartTool()
{
InstallNewInstance();
}
public override StatusInt OnElementModify(Element element)
{
return Bentley.DgnPlatformNET.StatusInt.Error;
}
private void CreateSuperElevations(Bentley.CifNET.GeometryModel.SDK.Alignment alignment)
{
ConsensusConnectionEdit con = ConsensusConnectionEdit.GetActive();
GeometricModel gm = con.GetOrCreateGeometricModel();
if (gm == null)
{
con.Close();
con.Dispose();
return;
}
con.StartTransientMode();
//Create SuperElevation Sections
string sectionName = "Section1";
double startDistance = 0.0;
double endDistance = alignment.LinearGeometry.Length;
string featureType = "SuperElevation\\Superelevation";
Bentley.CifNET.GeometryModel.SDK.SuperElevationSection superEleSection = gm.CreateSingleSuperElevationSection(sectionName, startDistance, endDistance, alignment);
if (superEleSection == null)
return;
//Set FeatureDefinition to the SuperElevation Section
bool status = superEleSection.SetFeatureDefinition(featureType);
//Create a SuperElevation Lane Right
Bentley.CifNET.GeometryModel.SDK.SuperElevationType superElevationType = SuperElevationType.Primary;
Bentley.CifNET.GeometryModel.SDK.Side superElevationRightSide = Side.Right;
string rightLaneName = "RightLane";
double insideEdgeOffset = 10;
double width = 10;
double slope = 0.02;
double laneStartDistance = 0;
double laneEndDistance = alignment.LinearGeometry.Length;
Bentley.CifNET.GeometryModel.SDK.SuperElevation superElevationLaneRight = superEleSection.AddSuperElevation(superElevationType,
rightLaneName, superElevationRightSide, insideEdgeOffset, width, slope, laneStartDistance, laneEndDistance);
//Create a SuperElevation Lane Left
Side superElevationSideLeft = Side.Left;
string leftLaneName = "LeftLane";
Bentley.CifNET.GeometryModel.SDK.SuperElevation superElevationLaneLeft = superEleSection.AddSuperElevation(superElevationType,
leftLaneName, superElevationSideLeft, insideEdgeOffset, width, slope, laneStartDistance, laneEndDistance);
if (null == superElevationLaneRight || null == superElevationLaneLeft) return;
con.PersistTransients();
}
}
}